To generate an iTunes library for consumption in F# using visual studio simply do the following:
1) create a new F# console application.
2) right + click references and add a new reference off the COM tab. We want the iTunes Type Library. I happen to have version 1.13 but you may have a newer version which is fine, go ahead an add the type library.
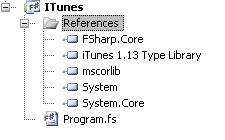
3) You can see from the screen shot above that the reference got added
and generated a typed library for us in the project bin or obj directory.
The name of the generated library is Interop.iTunesLib.dll
Now the fun begins, we can open a new F# script file
and dot into this library to see what it offers.
Below is the F# script file that I came up with.
#i @"path-to-dll-here"
#r "Interop.iTunesLib.dll"
open iTunesLib
(*** Grab a handle to iTunes ***)
let appcls = new iTunesAppClass()
(*** Play the current playlist ***)
appcls.Play()
(*** Shuffle the current playlist ***)
do appcls.CurrentPlaylist.Shuffle <- true
(*** Next Track ***)
do applcs.NextTrack()
(*** Previous Track ***)
do applcs.PreviousTrack()
(*** Back Track (Go back to the beginning of the track ***)
do appcls.BackTrack()
(*** Sound Volume ***)
do appcls.SoundVolume <-75
(*** Pause ***)
do appcls.Pause()
(*** Rewind ***)
do appcls.Rewind()
(*** FastFoward ***)
do appcls.FastFoward()
(*** Co-worker interruption ***)
do appcls.Mute <- true
//Lets have some fun with tracks
(*** Helper function to play a track ***)
let play (track: IITTrack) = track.Play()
(*** Print out All tracks in current playlist ***)
do
for item: IITTrack in appcls.CurrentPlaylist.Tracks do
printf "%s \n" item.Name
(*** Retrieve track by name & play it ***)
do appcls.CurrentPlaylist.Tracks.ItemByName "Too Much" |> play
(*** Just found out about a new FP Podcast ***)
do appcls.SubscribeToPodcast("url-of-podcast-here")
(*** What's New on iTunes!? ***)
do appcls.GotoMusicStoreHomePage()
A couple of things worth noting here.
1) iTunes needs to be open and running before you can manipulate it.
2) The above script just scratches the surface of what's possible with communicating with iTunes.
You can also convert files, tap into the browser and other operations
that you'd normally have to use the UI to do.
Personally I like staying in the fsharp interactive window. =)
-Develop with passion
No comments:
Post a Comment